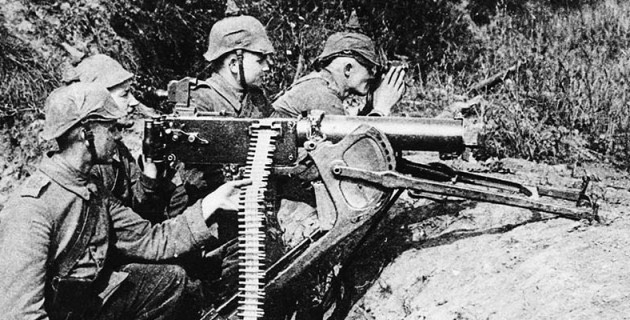
This is a machine gun in World War I. This weapon revolutionized warfare; it slaughtered millions. It was the perfect symbol for the mechanization of warfare. The machine gun is also an example of the looping process. It fires a bullet, then uses the energy of the recoil to pull another bullet into the chamber, which it then fires, and so it goes, over and over.
But there’s another critical element in this that is often overlooked: the belt of bullets that are fed into the machine gun. A machine gun without an ammo belt is useless; the ammo belt is just as important as the machine gun.
Loops in computer programs are exactly the same as machine guns; they need ammo in the form of data. The data must be laid out in a nice neat row like the bullets in an ammo belt. That nice neat row of data is called an array. A loop in a computer program chews through an array of data just like a machine gun chews through an ammo belt.
An arry is a ordered list of data. Here’s an example of an array:
x[0] = 23;
x[1] = 47;
x[2] = 19;
x[3] = 12;
…and so on. In JavaScript, you can have arrays of any kind of data: strings, numbers, booleans, and so forth.
You use a loop to step through an array, processing each member of the array in turn. Here’s a really simple example of an array and a loop that uses it:
for (var i=0; (i<4); ++i)
console.log(x[i] * x[i]);
}
This simple loop merely prints the squares of the numbers in the array.
You can use loops and arrays to process humongous amounts of information. You can address several different arrays inside a loop, combining their values to determine new results. Let’s use the capitals of Europe as examples:
capital[0] = Rome; latitude[0] = 41.9; longitude[0] = 12.5;
capital[1] = Paris; latitude[1] = 48.9; longitude[1] = 2.3;
capital[2] = Berlin; latitude[2] = 52.5; longitude[2] = 13.4;
capital[3] = Copenhagen; latitude[3] = 55.7; longitude[3] = 12.6;
This simple loop merely prints the squares of the numbers in the array.
A common operation in programming is finding the biggest or smallest result of some calculation. For example, let’s figure out which of these capitals is closest to Rome. We use a loop that calculates the distance from Rome to each capital, then compares it with the best result we have obtained so far. If we do get a better result, then we store that for the following comparisons. Here’s how the code works:
var closestDistance = 100; // We initialize this variable to a really big number
var closestCity = -1; // this insures that we start off with an impossible city index
for (var i = 1; (i < 4); ++i) // note that we start with index 1, since index 0 is Rome
var distance = Math.sqrt((latitude[i]-latitude[0])**2 + (longitude[i]-longitude[0])**2);
if (distance < closestDistance) {
closestDistance = distance;
closestCity = i;
}
}
Here’s a simple, easy challenge to show you how easy it is to use arrays.
This example shows you an even easier way to use arrays.