Contents
Elementary Arithmetic Methods
Chris Crawford
Triumph of the Individual
Greg Costikyan
The Self-Publishing Option, Part 3B
Kathy Crawford
Editor Chris Crawford
Subscriptions The Journal of Computer Game Design is published six times a year. To subscribe to The Journal, send a check or money order for $30 to:
The Journal of Computer Game Design
5251 Sierra Road
San Jose, CA 95132
Submissions Material for this Journal is solicited from the readership. Articles should address artistic or technical aspects of computer game design at a level suitable for professionals in the industry. Reviews of games are not published by this Journal. All articles must be submitted electronically, either on Macintosh disk , through MCI Mail (my username is CCRAWFORD), through the JCGD BBS, or via direct modem. No payments are made for articles. Authors are hereby notified that their submissions may be reprinted in Computer Gaming World.
Back Issues Back issues of the Journal are available. Volume 1 may be purchased only in its entirety; the price is $30. Individual numbers from Volume 2 cost $5 apiece.
Copyright The contents of this Journal are copyright © Chris Crawford 1991.
_________________________________________________________________________________________________________
Elementary Arithmetic Methods
Chris Crawford
Note written January 14th, 1998: This is quite dated stuff, but back then we really did sweat getting decent performance out of our 8-bit machines.
Time was when the amount of processing you had to do to support a game involved little more than some simple boolean operations to determine whether the bullet hit the target. We’ve come a long way since those days, and nowadays our games require far more complex computations. In this article, I’ll go over some of the elementary considerations surrounding the use of arithmetic in games.
The first and most important consideration is the choice of number representation for arithmetic calculations. There are four common options: 8-bit bytes, 16-bit words, 32-bit longwords, and floating-point representations.
Eight-bit representations went out with eight-bit CPUs. Those designers still stuck with eight-bit CPUs do not have many options. This article can do nothing for them other than request a moment of sympathetic silence from the reader. There is, however, one situation in which 16-bit designers might still wish to use 8-bit bytes; more on this later.
The 16-bit integer is now the primary representation for data in computer games. It is more than adequate for most purposes; under ideal circumstances, a 16-bit word offers average resolution of one part in 16,000, or better than .01%. In practice, there are quite a few gotchas to cope with.
First is the fact that the effective resolution of the 16-bit word decreases with the value used. Suppose, for example, that you want to record the wealth of a character in a game., measured in dollars. What happens if you have a rule penalizing errant players with the loss of half their wealth? If the character has 25,000 dollars, this works fine. But if the player happens to have 3 dollars, halving his wealth will give him 1 dollar; that’s quite an inaccuracy. It’s even worse if the player has one dollar.
A common solution to this problem is to constrain the dynamic range of the values accessible in the game. You come up with a brute-force rule that keeps the player’s wealth between a lower limit and an upper limit.
Underflow and Overflow
Two of the most common (and frustrating) problems are overflow and underflow. Overflow occurs when you push a number over its maximum permissible value. For example, if the player has a wealth of 32,000, and then finds a treasure trove of 769 dollars, the player’s wealth should be increased to 32,769. Unfortunately, a 16-bit signed integer cannot show a value in excess of 32,767. Therefore, when you add 769 to 32,000, you will get a result of -32767. Underflow is the same problem at the bottom end of the scale. If you take -32767 and subtract 769 from it, you will get a result of 32,000.
Artificially constraining a value to stay within a particular range does work, but it has its own problems. First, your code becomes inelegant as you backstop every calculation with "IF x > MaxLimit THEN x:=MaxLimit" statements. More important, it is artificial; one way or the other, the user is going to encounter the constraint and wonder, why can’t I have more than 32,767 dollars? Imposing artificial constraints on the user is bad form.
There’s a sneakier way to get around the problem. Instead of putting a hard ceiling over the head of the value, put a soft ceiling. To use the wealth example, instead of arbitrarily confiscating any wealth that might put the player over the ceiling, impose a kind of progressive income tax. That is, as the player grows wealthier and wealthier, you make it harder for him to acquire more wealth. Note that this is just as arbitrary as outright confiscation, but is not so brutal nor so obvious. The player never encounters a brick wall; instead, he encounters a progressively firmer spongy wall. It’s still a wall, but it’s a nicer wall.
There are other solutions. The simplest is to use a larger word size. If 16 bits aren’t enough, go to 32 bits. Current CPUs can handle 32-bit integer arithmetic directly with little additional time cost. Of course, 32-bit words consume twice as much memory as 16-bit words.
Scaling Problems
Geometric manipulations (in which you adjust a number by multiplying it by a fraction rather than adding or subtracting a value) are an important component of any designer’s basic toolkit. Such techniques are useful for modeling growth rates, combat results, and a host of resource management issues. But they can raise a hornet’s next of problems.
Suppose, for example, that you have a value that you need to increase by 1%. There are two ways to do this: you can divide it by 100 and add the result to the original value; or you can multiply by 101 and then divide by 100. (Remember, this is integer arithmetic so you cannot simply multiply by 1.01)
The first approach, dividing by 100, could result in underflow errors if the original value is small. For example,if the original value is less than 100, dividing by 100 will result in a value of zero. Increasing by 1% will have no effect. This can be catastrophic is you are using an algorithm that slowly increases the value of a number geometrically. Even if your value is greater than 100, the calculation will still suffer from significant round-off errors if the value is less than, say, 1000.
The obvious solution is to first multiply by 101 and then divide by 100. That way, the small number is scaled up and then scaled down. Unfortunately, this can yield overflow problems if you are using 16-bit integers and your original value is greater than 326. So here is our quandary: to increase a value by 1%, that value must fall between 100 and 326. Even though it’s a 16-bit integer with a normal dynamic range from 0 to 32,767, we have to keep it in the range 100-326 if we want to be able to increase it by only 1%. That’s a severe restriction!
Fortunately, there is a solution: doubling up the word size. If you need to change a value by a small percentage, you first double its word size (from 16 bits to 32 bits), then multiply by the numerator, then divide by the denominator. Then you truncate back down to 16 bits.
Another solution is to restrict the original value to 8 bits, multiply first to scale up, then divide down. This is one good reason to use 8-bit values. In many cases, the value itself doesn’t need much dynamic range, but it can be used in calculations with lots of dynamic range. If you start small, your word size ceiling looks a lot higher.
What if you original value is already a 32-bit value? You’re not quite out of luck. There’s one last option: floating-point representations. Most compilers readily support floating point arithmetic. Many game designers, especially those of us from the bad old days of 8-bit CPUs without hardware multiply/divide, blanch at the thought of floating-point computations in a game. Not only are floating-point values RAM-guzzlers (32 bits is common), but they also guzzle CPU cycles. The last thing you need in a game any game, no matter how relaxed in pace is to keep the user tapping his fingers while you’re crunching floating-point numbers.
The good news is, the situation is not that bad. The modern 16-bit CPUs with hardware multiply/divide can crunch through a goodly number of floating-point operations without bringing your game to its knees. The trick is to avoid their use in fast real-time calculations. For example, flight simulators are particularly vulnerable to arithmetic problems. The dynamic range of the many variables makes integer arithmetic inappropriate, yet the time costs of floating-point calculations makes them prohibitive. This is what separates the flight-simulation men from the boys: the cleverness to create adequate flight models that work with integer arithmetic.
Under what circumstance, should you use floating-point values instead of integers? There are two situations that demand floating-point numbers. The first of these is an extension of the fractional adjustment problem. If you need to perform a fractional adjustment, and the value of the fraction is determined at runtime, you cannot be certain that the multiply-up/divide-down technique won’t overflow. Floating-point numbers are just about the only way to perform this calculation without fear of error.
The other situation calling for floating-point numbers arises when you have a large set of coupled equations. It is usually possible, when using just a few equations, to merely scale your integer values to cover the dynamic range of the problem. But hand-scaling requires you to anticipate all possible values that might arise during play. While this is easy with 2 or 3 coupled equations, it gets tricky with 10 and impossible with 30.
_________________________________________________________________________________________________________
Triumph of the Individual
Greg Costikyan
Recently, we have heard much of the notion that the individual game designer is an endangered species. “As time goes on,” says the argument, “computer games become increasingly complex. No single person can be expected to have all the skills needed to produce a game that meets the market’s standards, or to complete development in a reasonable amount of time. Studios are the future: design teams in which designers, programmers, artists, and composers work together on every project.”
And indeed, the trend in recent years has been away from independent freelancers and toward in-house studios.
Computer games are, in many ways, far more difficult to realize than games in almost any other medium. The medium itself is refractory and difficult to work with. To make a change in a paper game may require retyping a page of rules, or whiting out a line on a map and drawing in a new one. Making a change in a computer game requires recoding; this requires a high degree of programming skill. And even the best of programming languages are not easy to work with, as anyone who has stayed up into the wee hours of the morning wrestling with bugs can attest.
Indeed, it is my contention that one of the reasons the average paper game is more satisfying than the average computer game (I make no such claim for the best of each genre) is that it is far easier to change paper games. The bulk of a paper game designer’s effort is spent refining the game and making it more playable and fun; the bulk of a computer game designer’s effort is spent merely getting the damn code to work, refining it, and getting it to work again.
And as computer games have gotten more complex, the computer game designer’s problems have compounded. Every little dippy bit of animation, which may engage the player for a maximum of sixteen seconds, requires its own code. Increasing quality of graphics, sound, and interface responsiveness place heavier and heaver programming demands...
But why should this trend continue?
From the game designer’s perspective, this is far from the ideal state. Ideally, we should be liberated from the drudgery of programming, and be able to craft our games in some “game space”, utilizing the power of the machine itself to turn our conceptions into executables.
But — wait a minute! Isn’t this exactly what the continuing development of computer science is supposed to do? Liberate us from the drudgery of our work, and allow us to concentrate specifically on the underlying creative elements? Word processing allows us to create documents without the tedium of typing repetitive drafts. Spreadsheets allow us to perform business projections without the drudgery of repetitive calculation. CAD/CAM allows us to design objects without redoing drawings and schematics. MIDI technology allows the composer to experiment with alternative phrasings, developments; to modify or reproduce his music at the touch of a button.
As time goes on, and as AI technology becomes more sophisticated, our mechanical helpers will perform more and more of the drudgery of everyday work. The mechanical secretary will take my calls, query the caller about his concerns, and pass important calls onto me while taking messages from others. The intelligent house’s motion detectors will see me getting out of bed, and so signal the coffee machine to grind some more beans and start a new pot. And when I sit down to my paper-quality flat-display with my steaming cup of Italian Roast Espresso, I’ll say, “Hello, Jeeves. I’m not happy with the combat resolution algorithm in ATTACK OF THE STAR SLAVERS. Could you recap it for me?”
What, after all, is a game? It is a set of data defining an initial state; a set of algorithms that act upon that data and other inputs; an interface to allow players to interact with the system; and a goal or set of goals that pit the players in competition, with the underlying algorithms and/or with each other. What we need is a “CAGED,” if you will: Computer Assisted GamE Design. In its initial stages, perhaps, it might include a “design painting program” that allows me to create data structures, then link them via algorithms — preferably using an easy, intuitive interface. Maybe it would look like this:
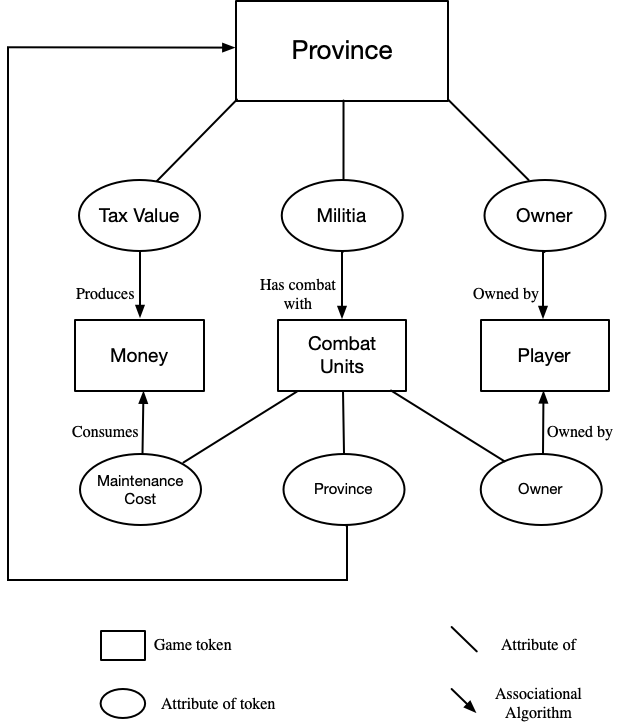
Once my structures and associated algorithms are established, I can assign each a screen representation. I can design an interface, preferably using standardized building blocks — hot keys, drop-down menus, pop-up boxes, etc. — simply by selecting from a list, plopping down appropriate control items and text, and indicating what event triggers the appearance of a particular item, and what data or algorithms the item allows the user to modify.
Graphics can be designed using whatever graphics package I wish. Similarly, sound can be generated using MIDI software, or by sampling real-world sounds and distorting them in appropriate ways. And, naturally, I can play the game in an interactive development environment, allowing me to make changes on the fly.
This, of course, would be only the beginning. Mice and keyboards are not particularly natural interfaces. Eventually, of course, we will want the use of natural language to control our machines; and we will want to be able to converse with our intelligent design assistants at a fairly high level. The machine ought to be able to suggest alternatives, refer to its memory of existing games and offer analogies, call online services to help us do research, generate graphics from verbal specifications and modify them as directed. It ought, in short, to be an intelligent assistant, turning us from keyboard jockeys into true designers: people who spend their time designing and not worrying about the details of implementation.
In general, as computer technology advances, software tools make it possible for everyone to do things that used to be the domain of specialists. The same is likely to be true in software, as in every other domain of human endeavor; indeed, it had BETTER be true, because otherwise the complexity of the machines with which we deal and the complexity of the demands we place upon them will rapidly outpace our ability to generate the necessary software.
Consequently, as time goes on, it will become possible for people with fewer and fewer programming skills — but with the ability to envision and define interesting games — to create workable systems. The quality of computer games will improve, as people with talent but without knowledge of the arcane details of bit-twiddling are empowered to develop games.
And, two or three decades hence, I expect we will see in computer gaming roughly what we see in the field of publishing: a horde of independent creators, selling their product through a number of publishers, few if any of which maintain their own, expensive, in-house staffs.
_________________________________________________________________________________________________________
The Self-Publishing Option, Part 3B
Kathy Crawford
In the last article, I discussed options for Customer Service. This article assumes that you have reviewed your options and decided to set up a customer service department as part of your new publishing business. This is a substantial long-term commitment that can pay off or bankrupt you. Let’s discuss the costs of three areas: Facilities, Utilities, and Employees. These will give you an idea of what to expect.
Facilities
Finding space for your business can be as easy as setting up another desk in your spare room. I know of a two-man company (owner and one employee) that does operate out of the owner’s guest room. This is a cheap option, if you have a guest room and think that you could stand having employees in your home. Most of us will need or want to rent office space. For two to three people, you will need 500 to 1000 square feet. Offices of this size normally exist in an office complex rather than a separate building. I found our office by driving around the area in which I wanted to locate and calling all of the " Office Space For Lease" phone numbers I found. Out of eight office complexes, there were three offices in the under 1000 square foot size range. That’s not very many, but they do exist. The office is your first commitment. In San Jose, the going rate for small office space is about $1.40/square foot/month That is $700/month in rent for a 500 square foot space. Most offices are leased. In San Jose, you have to negotiate pretty hard to get a two year lease, the standard is three years. Two years at $700/month is a commitment of $16,800. There are other details about leasing that you will learn after you talk to a couple of realtors. Just make sure you know the entire cost of your lease before you sign.
There are other space options to explore. Sometimes an office will be rented month to month. This is a seductive option because if you get into trouble you can shut down quickly. BEWARE! The owner can shut you down just as quickly. I know of a company that was kicked out of its space during a critical time. The owner lost a month of productivity while she looked for new space and then had to accept the best she could find on short notice. Also, if you publish your customer service telephone number, your new space will have to be in the same telephone prefix area in order for you to retain your phone number. Sometimes the prefix area can be a few square blocks.
Another option to consider is subleasing space in an existing business. Many businesses rent space to grow into (or contract in hard times). This has the advantages of a small space and the possibility of providing secretarial, fax, or copy services as well. However, the same cautions listed above apply in this situation. When you outgrow the space, can you move in the area so that you keep the same customer service number?
Utilities
Deciding what utilities you need is easy once you decide what your customer service representatives will be doing. First, there is the phone. You will need to print your customer service telephone number months before your first call. With Pacific Bell, we were able to tell them the area where our office would be located, have them assign a number, start paying a small monthly fee, and then have the line installed two months later. They would not hold a number for us for free, but the uninstalled monthly fee was small.
Second, to what address will your warranty cards be mailed? I recommend a post office box. If you publish your office address, you are limited when the time comes to move. Sure the post office will forward your mail. Sure... A post office box will cost about $50/year and take about eight weeks to get. Also, the post office will create artwork for your warranty card return address, including their special postal bar code. I haven’t tried it yet, but I think that you can get this service for free.
What do you do with the warranty cards that you receive? Before you can answer that question, you must decide what their purpose is in your business. Warranty cards are the truest measure of sell-through. The return rate ranges from 3% to 20% and once you ascertain your return rate, you can watch the sales of your products on a weekly basis. But there’s more information to be derived from warranty cards.
Look at the warranty cards from several publishers to decide what information they think is important. Name and address is standard. We included a number of check-boxes for the hardware configurations of our customers. Some publishers include demographics, such as age, income, or sex, and product rating questions. The cards are a bother for customers, especially if they have to put a stamp on the card, so make sure that you are only asking for information that you will use. That will increase the chance of your customer filling out and mailing the card. We also serialized the cards. I think that this increased the return rate.
So warranty cards can provide you with a mailing list, survey information, and registered users. But to use the information, you will need to enter the information into a data base. You can create your own, but I recommend you buy one. We use "AnswerSet Call Tracking System" from AnswerSet Corporation (21771 Stevens Creek Blvd., Cupertino, Ca 95014; 408-996-8683). I highly recommend this program. The warranty card information is entered into the data base and each incoming call is also entered. The system has some default reports and you can write your own custom ones as well. Some of the reports we get out of the system are sales by state, hardware configurations of our customers (from the check-boxes on the warranty card), number and type of calls by SKU, and mailing labels of our educational customers so we can send them additional information. There is even a report called the logbook that reports those calls that you have flagged as important. A new customer service person can read this and get a good idea of what calls to expect and how to answer them. The AnswerSet system can also be networked to grow as you grow. It costs $995 for a single station, but it’s worth it.
Employees
People are the most expensive part of customer service, in every way. There are many books on the legality, ethics, and emotional aspects of management. I will confine myself to some of the basics and save my anecdotes for another time.
Before you hire your first employee, you need to register with the IRS and probably your state for proper withholding of income tax. You will need to file a form to get a Federal ID number and, in California, with the EDD to get an EDD number. After you hire your first employee, they will have to fill out a W-4 and I-9 form. Does this sound complex? It is. I advise you to find a payroll service. It is the best $50.00 I spend every month. They write the paychecks to my account and send them to me to be signed and delivered. They figure the withholding for the various taxes, pay the taxes for me (from my account of course), and file the quarterly returns. Shop around; the prices and services really vary. You can start at your bank because many banks have a payroll service as part of their business. Most of these services charge by the payroll run, with a small per check charge, so that a monthly payroll will be cheaper than a weekly. I found every two weeks to be optimal for me and my employees.
Also, if you live in California, you can call the California Chamber of Commerce (800-331-8877) and order a "Business Start-up Kit". It is a book of forms to help you get going. It costs about $30.00 and there is a different set for individual proprietorships, partnerships and corporations. All of the forms I mentioned here are in that book with instructions, phone numbers, and addresses. If all of this sounds overwhelming, just get your spouse to do it -- like Chris did.
_________________________________________________________________________________________________________
The 1991 Computer Game Developers’ Conference
Editor’s Note
The 1991 Computer Game Developers’ Conference was bigger than any previous conference. We had 550 registered attendees over the two and a half days of the conference. It was, by all accounts, a very successful get-together. There were some problems: our choice of an open-air setting backfired badly when a big rainstorm pelted us with showers. And our neat idea of having pizza and hamburgers for lunch didn’t turn out to be quite so clever when confronted with the gustatory reality.
Because the conference was so big, it is impossible for one person to capture the experience of being there. I therefore asked a variety of people to provide me with vignettes from the conference. Here are their contributions:
Bill Pirkle
The fifth annual CGDC! The first CGDC was held in a living room and now over 500 people attend this three day cram course in everything from computer game design fundamentals to self publishing - the entire spectrum of entertainment software production. But among this cornucopia of jewels of technology were two diamonds. The Software Publishers Association generously provided an abundance of information about the computer game industry. Figures on sales of games by genre and platform and figures on game sales over time by genre help in tracking that moving target - the future course of the computer game industry. Tracking the public’s artistic interests is made easier with these data and they were enlightening. Enlightening also was the information from the session on the “State of the Entertainment Software Industry”. This diamond, too, cast a white light of truth about the industry from another dimension and in a clever way making many points effectively. Accordingly the two sessions combined to bring the industry more sharply into focus. Dependably, facts revealed at sessions like these spawn ideas which offer solutions to problems as the computer game industry follows an enthropic course toward an increased state of order and continues its evolution into a form which survives in today’s highly competitive international marketplace. I hope that next year we will see even more.
Ken St. Andre
I don’t attend many high-priced computer conferences, but for the Computer Game Developers’ Conference this year I made an exception. (Getting to go as staff brought it down into my price range.) The CGDC is specifically a conference for those people who are actively involved in the creation of computer games of any sort — cartridge, floppy, online, CD-Rom, and by next year they will probably be into virtual reality as well. The computer gaming press is also represented as there were people there from Compute Magazine, Computer Gaming World, and a Japanese magazine whose name I can’t pronounce. Some of the larger computer game corporations, such as Lucasfilm and Origins support the conference; some such as Sierra don’t. This year the conference was held from March 10th through 12th at the Hyatt Hotel in San Jose, California, and in its fifth year of existence had its largest attendance yet — about 550 people.
I arrived on Saturday around noon and spent the early afternoon talking to people, doing a few chores, and getting my instructions on what to do as a Conference Associate. Some CAs had the difficult chore of managing registration tables, but there were so many volunteers for those positions, and I had other things to do, that I missed that part of the work. Mostly my job was to set up signs, count heads, and turn on tape recorders. All in all, it was easy work, and the conference t-shirt that I got for my efforts was adequate compen-sation for the little labor I actually contributed. (a quality t-shirt in heavy cotton with the motto “Cogito ergo ludo” on it in pop-art lettering.) Conference t-shirts are an annual project of Jeff Johannigman who is now a producer for Dynamix, but last year was the producer for Savage Empire and Wing Commander from Origins. I managed to spend a few minutes talking with Chris Crawford before things really got started, and in a quiet way that was one of the better parts of the three days for me.
For several years now my prime reason for attending conferences and conventions has simply been to see people — to renew old friendships and make new ones. While some people go to these things because they have products to sell, or people to hire, or speeches to give, I’m there just to keep up with developments in gaming and to talk to friends. Such networking is also a good way to get assignments if one is a free-lancer like myself.
Although there were several notable talks and presentations at DevCon this year, some of which I was fortunate enough to attend, the high points of the convention all came at supper time for me. On Saturday night, while most convention attendees were going to the huge schmooze, supper, and party that Apple Computer threw at the Hyatt, I joined some 19 other people and went off to Benihana’s in San Jose for the GEnie SFWA supper get together. This is something that I started by saying I’d like to meet and eat with other Science Fiction Writers of America members who might be in the San Jose area. Some computer people like Mark Baldwin, Corey and Lori Cole, Jeff and Stephanie Johannigman, and Eric Dybsand joined a lot of non-computer people for an excellent supper beyond the Hyatt, and there were hours of good food and laughter, very little of which had anything to do with computer gaming. I liked it because I met people that I had been talking to on GEnie for over a year.
The second supper was an impromptu GEnie JCGD meeting thrown together hastily by Dave Menconi and Angela Jones. About 20 GEnie regulars met at the DevCon suite and then took off on Sunday night to find food. It was good to fit names to faces. That group broke into two parties. I don’t know what happened to one of them, but the group I was in that included both Dave and Angie wound up at the Red Lion Inn about two blocks away.
The third great meal was the banquet on Monday night. All 550 of us attendees were there for that one. I quite enjoyed my chunk of “dead cow”, and was able to sweet talk our table waitress into bringing me a second piece of the delicious chocolate trifle they served for dessert. Eight awards were presented after the banquet. Unfortunately, the slide show accompanying the awards presentations showed only the title screen from each game nominee, but even at that it gave people something to watch, other than the speakers who were all Conference directors, and was quite successful. (A list of the eight award winners follows this essay.)
The other high point of the banquet was the Guest of Honor speech by cyberpunk science fiction writer Bruce Sterling. To tell you that his talk, which compared computer games to science fiction writing, computer media to books, and game designers to SF writers was howlingly funny is a pale understatement of Bruce’s eloquence, but, in fact, I was too busy laughing to take notes, and cannot reproduce any of his lines. I do recall that Bruce said it wasn’t the wonderfulness of storytelling that carried sf novels and games — it was the weirdness, and that as designers we should embrace our weirdness, and be the geekiest computer geeks that we could — that way lay the path to true greatness. What a speaker! To call us all a bunch of geeks and nerds and make us like it!
There were other fine moments at the conference for me. Computer Gaming World threw the most successful party of Sunday evening just across the hall from my room at the hotel. Thanks for the wine and cheese, Johnny and Russ! I would have liked to have stayed and talked more, but your room was so crowded that I couldn’t breathe in there. Then there was Chris Crawford’s talk on the “Evolution of Taste”. He talked about the four C’s: candy, cartoons, comics, and computer games. They are all intense, brightly colored, fun-fun-fun, and appeal primarily to children. If Chris ever gets tired of designing computer games for a living, I’m sure he could make a good living as a demagogue comedian. What a great talk . . . and it did make one think about how computer games are evolving (or not evolving).
I must have met 20 or 30 people at the convention that I had never met before. Although the cost of attending for most participants varied between $200 and $300 plus hotel bills and transportation costs, the Con Committee did such an outstanding job of providing food for both mind and body that I’m sure most people thought it was worth every penny. I came to the Con to meet and talk to people, and I was able to do so in grand style. A list of the people at the Con that I spent some time with would fill the rest of the page, so I’m going to skip it. You know who you are!
If you have any interest at all in computer gaming, I heartily recommend that you make plans to attend next year’s conference scheduled for April 26.
Winners of Developer Awards
Best Gameplay
Secret of Monkey Island
Best Interface
Loom
Best Graphics
Wing Commander
Best Audio
Wing Commander
Best Producer
Greg Hammond/Lucasfilm
Most Socially Responsible
Sim Earth
Most Innovative
Sim City
Best Technical Achievement
Wing Commander
From “An Observer”
• The 1991 Computer Game Developer’s Conference was held at Nome, Alaska.
• The unofficial theme of the conference was established at the bittersweet Roberts/ Crawford debate, “Presentation vs Game Play.” Chris Roberts never had a chance. Expecting a room full of game designers to admit that sizzle is more important than steak is like expecting the Academy of Motion Picture Arts and Sciences to admit that E.T. was 1982’s Best Picture. The sporting gentle-man from Texas will have to find solace in the plaques he took home from the awards banquet. Perhaps the quarter million sales Wing Commander is likely to attain will offer some comfort as well.
• More wannabees than ever this year. I met one young hopeful who drove all the way from Missouri to attend. Heartbreaking. We must do everything we can to help these people break in, sadistic as this may seem.
• Monday’s lunch (hot dogs and microhamburgers) was awful. Sunday’s lunch (cold pizza) was TransAwful. The banquet dinner was decent; perhaps it was shipped in from another hotel.
• There was no publisher’s panel this year. It was feared that one of the participants might go out of business during the session, or that two or more would start merging in front of everybody.
• Lucasfilm sent twenty-six employees to the Conference, more than any other company.
• Lucasfilm appeared on the final awards ballot eight times, more than any other company.
• Origin won three awards, the same as Lucasfilm. They sent three employees.
• A pair of Learning Company educational games appeared on the final ballot. Nobody I asked had ever heard of these games.
• The Learning Company sent seventeen employees to the Conference.
• Nominee Dan Bunten reportedly left the banquet hall when the awards ceremony started.
• The youthfulness of many conference attendees was reflected in the fact that Star Raiders did not win the award for Best Technical Achievement.
_________________________________________________________________________________________________________
The Evolution of Taste
Chris Crawford
Candy
Consider candy. Candy is fun food. What makes candy so special among foods? I think that it’s because candy is intensely pleasurable. Have you ever noticed just how intense an experience candy provides? It doesn’t taste merely good; candy makes your tongue jump up and shout with joy. It is an intense experience. Now, there are other intense gustatory experiences -- chili peppers, for example. But they are not intensely pleasurable. A chili pepper makes your tongue scream, not laugh.
The intensity of candy has some consequences. First, candy must be taken in small doses. You don’t eat an entire meal of candy, just a small piece.
When I was a child, I loved candy. It was my favorite food. But as I grew older, I became bored with the taste of candy. I wanted more subtlety and more variety in my eating experiences. By the time I was a teenager, my tastes had matured to favor such sophisticated foods as hamburgers, pizza, and hot dogs. As I grew older, my quest for subtlety and variety led me to try Chinese food, different breads, barbecue sauces, Italian food, cheeses, seafoods, salads all manner of foods.
The aggregate efforts of millions of people pursuing similar courses has spawned a huge gustatory universe populated with a staggering variety of culinary delights: Thai food, peanut butter, wines, T-bone steaks, Béarnaise sauce, blackened foods, caviar, dill bread, and on and on. And one small subset of this universe is the world of candy, characterized by several traits: it is fun, intensely pleasurable food, taken in small doses, and primarily appreciated by children.
Cartoons
Now consider cartoons. Cartoons are the most fun form of video. Sure, I enjoy many forms of video, but cartoons make me laugh more. What makes cartoons so much fun? I think that it has to do with the fact that they are intensely pleasurable. Look at the colors in a cartoon: all bright, loud colors no soft pastels or delicate shades here. Or consider the pace of a cartoon. Everything happens at breakneck speed. Characters dash about frenetically, never giving the viewer a second to catch his breath. And there’s nothing subtle about danger in cartoons. Characters are assailed by falling safes, flocks of flying knives, sizzling sticks of dynamite, falls from cosmic heights.
Herein lies some of the pleasurable aspect of cartoons, for the characters are never seriously hurt by all this mayhem. Explosions merely blacken their faces. Falls from great heights produce body-shaped craters from which the character emerges, to wobble away unhurt. The impact of a falling safe flattens the character, who peels himself up from the ground to reinflate his body as if it were a balloon. This disjunction between terrible danger and lack of serious harm is a pleasurable release; it is fun.
The intensity of cartoons requires them to be short, only a few minutes’ duration. This point is exemplified by the movie Who Framed Roger Rabbit? which started with a magnificent cartoon lasting all of three and a half minutes. Then the cartoon transformed into a movie: the bright colors softened, the pace slowed down to that of real life, and the intensity dropped down to a level that could be sustained for two hours.
When I was a kid, cartoons were my favorite form of video. I’d watch them all day long, if I could. But as I grew older, I became bored with the sameness of cartoons. I longed for characters who were more than cute little animals. I wanted some conflict that was resolved with more subtlety than a mallet-blow to the head. I wanted more variety and more subtlety in my video. So I began to watch more sophisticated programs, programs like Gilligan’s Island and Lost in Space. And later still, I started watching even more serious video, so that now my viewing habits include the nightly news, Connections, and movies like When Harry Met Sally, Out of Africa, and Koyaanisqatsi.
Most of us followed a vaguely similar path, and now our combined tastes have created a huge universe of video pleasures, including comedies, how-to shows, mysteries, game shows, pornographic movies, talk shows, action-adventure shows, soap operas, kiddie shows, and many more forms of video. Cartoons comprise a small subset of this gigantic universe, distinguishable by the fact that they are fun, intensely pleasurable, taken in small doses, and primarily appreciated by children.
Comics
Consider comics. (For the purposes of this discussion, I shall exclude from consideration the serious comics of recent years, comics such as Maus. Instead, I shall consider only the mainstream comics.) Comics are fun; I get a kick out of reading them. Comics are fun because they are intensely pleasurable. Look at the drawing style in the comics: bold, clean lines, with no hint of subtlety. The colors are bright and pure. The characters, conflicts, and events in the comics are always intense. Good guys are as good as they come; bad guys are ugly, deformed, and truly evil. The good guys always win; that’s one of the things that makes comics fun.
Comics always come in small doses, largely because their intensity cannot be maintained over a long time. Their first audience is children. When I was a kid, I used to read comics all the time. After a while, though, I grew bored with the sameness of comics. I wanted more variety, more subtlety in my reading. So I began to read more mature fare: Jules Verne and Mark Twain. Later I graduated to Thoreau, Hemingway, and Shakespeare. Now I read Toynbee, Braudel, and von Clausewitz.
Each of us has pursued a similar evolution, starting with comics and proceeding to more subtle literature. Jointly, our courses have spawned a gigantic universe of literature, with newsmagazines and science books, cookbooks and sci-fi novels, the National Enquirer and Playboy, dictionaries, car repair books, economics textbooks, devotional literature, and even The Journal of Computer Game Design. And way over in one corner of this universe is a tiny subset of literature known as comics, unique in that they are fun, intensely pleasurable, taken in small doses, and especially appreciated by children.
Computer Games
What do candy, cartoons, and comics have to do with computer games? Fun, for one thing. We all know that computer games are supposed to be fun, right? And in fact, computer games are intensely pleasurable experiences. Look at the visuals used in computer games: lots of bright colors. Subtle shades or elegant brush strokes are not appreciated by computer game players. Consider the animations: an emphasis is placed on big, bright explosions.
It goes far beyond the graphics. Consider the nature of the conflict: the player is always a good, good, good guy trying to save the universe from a bad, bad, bad guy. Whether it’s an Evil Wizard, a Vile Gangster, or merely the standard Evil Galactic Empire Bent on Galactic Domination, the basic relationship between characters is simple and intense.
The conflict between the characters is always resolved in the most intense manner possible: through violence. The violence in computer games is not attributable to any moral depravity on the part of players or designers; it is simply the most intense expression of conflict available.
This is pleasurable intensity because the player is expected to win. The player knows that he is supposed to win, that good will overcome evil, and that the game will have a happy ending.
So the analogy between computer games and candy, comics, and cartoons seems on the mark. But there are a few discrepancies remaining. For example, why aren’t computer games as closely associated with children as candy, cartoons, and comic books?
The answer has to do with the difference between age and experience. I moved on to more subtle foods not because I grew older, but because I grew more experienced. The act of eating several tons of sugar taught my palette to appreciate less intense tastes. Analogously, the act of playing several thousand hours worth of computer games has refined my tastes. Of course, I am atypical. Millions of adults have never experienced computer games; they constitute a pool of new players that have kept sales high. We must remember that this is a one-shot situation. Before long, we will have exhausted our pool of naive adult players. What will happen then?
We would expect these players to follow the same evolutionary path that we all followed with candy, cartoons, and comics. Specifically, we expect them to seek out more subtlety and more variety in their gaming experiences. We would expect them to move out into the larger universe of computer games. But what does our player find when he does so? Nothing. He finds an empty universe. Our player is an astronaut floating alone in a vast, dark universe with no stars, no galaxies, nothing. Where are the games to appeal to his more mature tastes? Where are the games that are analogous to Caesar salads, nightly news, or bodice-ripper novels? Where are the bagel-and-cream-cheese games, the Archie Bunker games, the Jacquiline Susanne games? Where are the games about a boy and his dog or the prostitute with a heart of gold?
I fear for the future of our medium. We all sit huddled in one corner of this universe, the fun corner, crowded together in clammy clannishness. We know how to make FRPs, flight simulators, wargames and graphic adventures, so that is all we do. We’re afraid to venture out into uncharted territory; after all, we have bills to pay. So we crowd together in familiar if well-trampled terrain.
While we dawdle, our audience continues to evolve. Their tastes change and, finding nothing in our universe to satisfy their new tastes, they write us off as children’s entertainment. With each passing year, the culture’s impression that computer games are nothing more than cheap thrills for kids becomes more firmly established. The day may come when we attempt to move out into the larger universe and find a barrier placed in front of us, a Tholian web of audience expectations that locks us forever into our tiny, fun corner of the universe. We may find ourselves as clowns who are never permitted to remove the paint from our faces, forced to laugh and pratfall for eternity. Today’s heaven could become tomorrow’s hell.
Perhaps our future is not so bleak. There are others interested in interactive entertainment, people from television, theater, and literature. They are not as crass as we are, and they are more willing to move out into the larger universe that we shun. Perhaps they will colonize the New World. Our greater experience in interactivity will of course be of value to them. Perhaps, when the credits roll, we will have a place somewhere between Property Master and Chief Gaffer.
One thing is certain: taste evolves. Its evolution rolls onward, remorselessly crushing any who cannot keep up.
Long-term evolution of taste
An excellent example of the evolution of taste over a long time span is provided by the Arthurian legends. These legends began during the nadir of civilization in the Dark Ages. In their earliest incarnations, they were much like candy, comics, and cartoons: they were intensely pleasurable. The themes were simple: Arthur as good guy takes on all manner of bad guys, and resolves the conflict through the most direct expedient: violence. The earliest versions of the legends are a dreary recital of all the people and monsters that Arthur slaughtered. The similarity between Geoffrey of Monmouth’s History of the Kings of England and a modern videogame is striking, only his stories are hack-em-ups rather than shoot-em-ups. Just as with candy, cartoons, and comics, they were taken in small doses: the legends were told by traveling storytellers who would tell one story each evening, taking several weeks to spin out the entire cycle of tales. They had to make a buck, too.
By the twelfth century, the French jongleurs had gotten ahold of the legends, and they began to change. The amount of hacking (intensity) diminished and they began to show greater variety and subtlety. More characters were introduced. Women began to enter the legends, at first for little more than providing excuses for hacking, later on as sources of romantic interaction, and soon afterwards as characters in their own right. In the fifteenth century Mallory introduced a synthesis of the legends that included romantic chivalric, and spiritual elements -- although the hacking component was still, by modern standards, overbearing.
The Victorian era saw a renaissance of the Arthurian legends, with new twists put on the old workhorse. Victorian values of honor, duty, and romance were expressed in this new round of the legends -- and Mark Twain used the same material to create a biting social satire. In this century, we have seen the legends used for standard Broadway romance (Camelot), wholesome Disney family fun (The Sword in the Stone), and even feminist self-assertion (The Mists of Avalon). The evolutionary path from intense pleasure (good guy hacks bad guys) to greater variety and subtlety has been adhered to throughout the 1200-year development of these legends.
_________________________________________________________________________________________________________
A Graphics Trick
Chris Crawford
A Fragment of Code
When I started at Atari back in 1979, they had a little test they used to challenge programmer candidates. They showed them this little fragment of 6502 code:
LDA FIRST
EOR SECOND
AND CONTROL
EOR SECOND
STA OUTPUT
Then they would ask the candidate what the code does. It seems that only the best programmers could figure it out. I don’t rightly recall whether I passed the test, but I do remember that code, because it is one of the sneakiest bits of code I have ever encountered. What it does is to build up a byte out of bits from both FIRST and SECOND, using CONTROL to pick the bits. That is, if bit #3 in CONTROL is a 1, then the value of bit #3 in OUTPUT will be equal to the value of bit #3 in FIRST. If bit #3 in CONTROL is a 0, then the value of bit #3 in OUTPUT will be equal to the value of bit #3 in SECOND. Thus, if CONTROL = 0, then OUTPUT = SECOND, and if CONTROL = $FF, then OUTPUT = FIRST. But these are uninteresting cases. The interesting case is when CONTROL is some value between 0 and $FF, in which case OUTPUT is a function of both FIRST and SECOND.
What’s it for?
At this point, you may be wondering, what is the point of this truly obscure fragment of code? I may not be the world’s greatest programmer, but I instantly grasped the significance of this code fragment. This is graphics manipulation code par excellance. This code permits magical things to happen on a computer screen. It enables you to mix two images on a screen in a variety of ways controlled by the complexity of the CONTROL parameter.
Here’s a simple example: suppose that you want to do a horizontal wipe from one screen to another. (A wipe replaces one image with another in an animated sequence that takes about a second. The new image wipes over the old one along a moving front.) The conventional approach would involve writing selected bits in the screen bitmap. (For the purposes of this article, I shall assume simple black and white displays. To extend these concepts to color displays, add bit depth to the examples.)
The basic algorithm
To explain the concept, I shall ask you to imagine three data structures. The first is the original bitmap on the screen, which I shall call FIRST. The second is the new bitmap that we intend to replace it with, which I shall call SECOND. The third bitmap is the control bitmap, which I shall call CONTROL.
The basic algorithm consists of two nested loops. The inner loop calculates a single screen; the outer loop handles all the screens in the complete wipe. The inner loop executes our magic little code fragment on each of the three corresponding bytes from FIRST, SECOND, and CONTROL. It then sends the resulting byte to the screen. This loop does this for all the bytes on the screen.
The outer loop manipulates only the values in the CONTROL bitmap. We first set the bits on one edge of CONTROL to 0’s. Then we set more bits in CONTROL to 0 and repeat the process until CONTROL is all 0’s.
Hotshot programmers will immediately dismiss this technique as impermissibly slow. After all, why waste cycles moving bytes that don’t change? You only need to change the bytes in a vertical column, so why go to all this trouble?
Extensions
Ah, but there’s so much more! You want to do a diagonal wipe? Just change the way you modify CONTROL. The inner loop remains the same. You want a more complex wipe? No problem! Anything you can draw into CONTROL can be the basis of your wipe. For example, suppose that you want a radial wipe. Start with CONTROL being all black, then, in the outer loop, paint a small filled white circle into it. With each iteration of the outer loop, paint a larger filled white circle into CONTROL. Continue the process until the screen is completely white.
This basic approach can be used with ANY geometry. You can create a huge number of wipes, and they don’t have to be specially designed and coded. All you modify is the outer drawing loop into CONTROL; the inner loop remains the same.
Dissolves
But there are other possibilities. One of my favorites is the dissolve. A dissolve fades one image into the other. In a wipe, the new image starts in a small portion of the screen and expands to fill the screen; in a dissolve, the entire image slowly emerges over the entire screen.
To execute a dissolve, you simply start with a CONTROL bitmap that is all 1s, and then start populating it with random 0’s. You can use different patterns for populating with 0’s to achieve different styles of dissolve. For example, you can use a simple procedure in which you repeatedly AND random numbers into CONTROL to get a clean dissolve. I found that it looks better if you replace each CONTROL bitmap with a new CONTROL bitmap; this makes for a more sparkly dissolve. This was the technique I used in Excalibur (1983) and later in the title screen for Balance of Power (1985). The worst problems with this technique involve getting balanced numbers of bits in the bytes you use for CONTROL. I had to build big tables of semi-random numbers with the correct numbers of bits set for each iteration of the outer loop. Thus, I initialized an array SEMIRANDOM[1..15] OF [1..32] OF Integer. This gave me 32 random numbers of each type, one type containing a specified number of bits set.
Speed considerations
How fast is this technique? Not fast enough to handle an entire Macintosh screen, even in assembly language -- although even a simple full-screen blit is too slow for most animations. Its use is necessarily restricted to subsets of the screen, and small subsets at that. I found that dissolving bitmaps only 128x128 required recourse to assembly language. My guess is that, to use this with pure CopyBits calls in the Macintosh, you’d need to restrict yourself to bitmaps smaller than 64x64. With assembly language you can’t quite make 256x256 fast enough.
On an IBM, the situation is trickier. First off, color makes everything run more slowly, and the algorithm is more complex. Second, you may be required by marketing considerations to support slower processors. Considerable experimentation and judgement will be required.
_________________________________________________________________________________________________________